Portals testing with Cypress.io
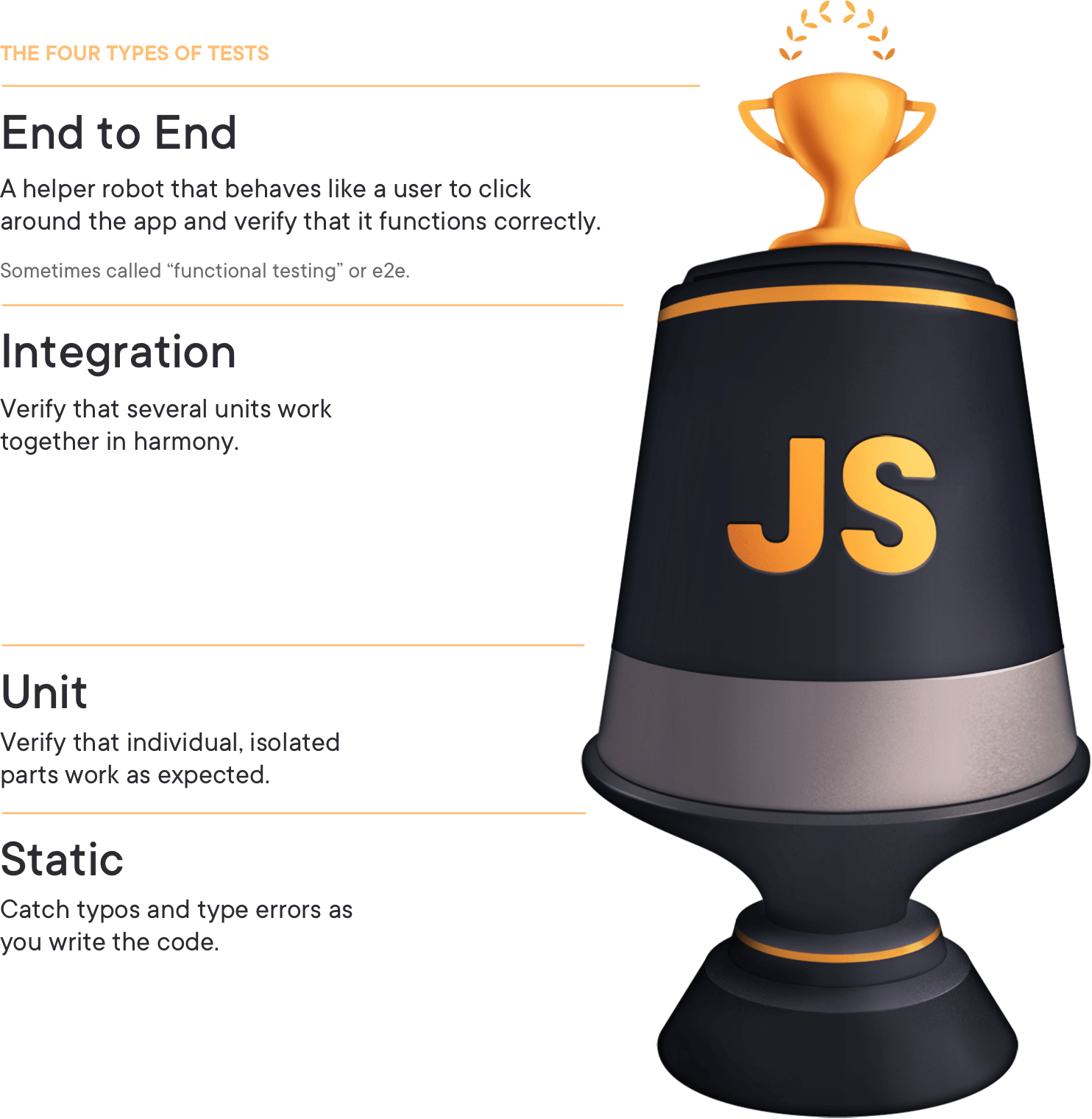
This picture sums up the types of test that you can perform for your application. You can start from simple basic tests such as static, covering them with tools like ESLint or Prettier, and then move to more in deep tests like End to End Testing (E2E). It’s always good to have a bit of them in your application, however, don’t over exceed with them, since too many tests are nonsense if with a few you are covering 100% of the errors you could have.
Today we are going to talk about E2E Testing inside Portals, and for that task, we are going to use Cypress.io. It has such awesome documentation and it is quite simple to follow. If you have used Selenium at some point in your life, these tests are going to be almost the same and it will vary in some aspects of the syntax but it is extremely powerful.
As a quite personal opinion, why I recommend you to perform tests when nobody likes testing? I will sell you this way, as a developer, what would you prefer, clicking a,b,c,d,e button or writing some code once and that could be repeated itself with no effort many times?
You need to sell your boss as “Testing is not a waste of time, is an investment for saving it for you and your co-workers.”
Cypress
But, what is Cypress? Cypress is the tool that we are going to use for our E2E Testing. This type of testing is 99% of your time performed by yourself, (if you haven’t used a similar tool like this). Yes, what I mean is, this tool will perform the same testing that you do when You fill the username with ‘victor’ then you fill the password with ‘myferpectsecretpassword’, then I click inside the submit button and finally I hope I see the main page with a ‘Hello Victor’
E2E Testing gives you that confidence and it will perform the same steps that you are doing manually in an automated way to save you that time you can spend in some other and more important tasks.
However, why nobody uses E2E testing? There are a few reasons, they are quite slow, you need to perform them with your computer opened and they are “expensive” to write. On the other hand, they give you that confidence and they save you that time that you could be performing the same task over and over again.
I will cover a scenario that you will be quite familiar to:
You create a new web page with some HTML and some Javascript, then you need to go to the _services/about to perform a Portals clear cache, then you need to go to your main webpage and go to that web page an validate that your Javascript is working.
What if I tell you these steps can be completed with Cypress, and just by pressing a button?
Installing Cypress
I would really suggest if you go to Cypress documentation and follow some of it if you want to get more familiar and you want to perform a clean installation.
Cypress installationI will start from the very beginning, as if we didn’t have any project or anything to start with.
First, let’s go to the path we want to install this:
cd ../yourpath/portalscypress
NOTE: Don’t call your package.json name as the dependency you want to install, for instance, don’t call the package as cypress
npm init
We set all the items as we would like and then we install cypress
npm install cypress --save-dev
We will need to wait a few moments so cypress is downloaded. The screen you will be getting will be something like:
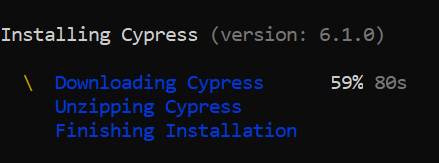
Once it is installed, let’s open your favourite IDE, I will be using VSCode for this
code .
We will have something like this:
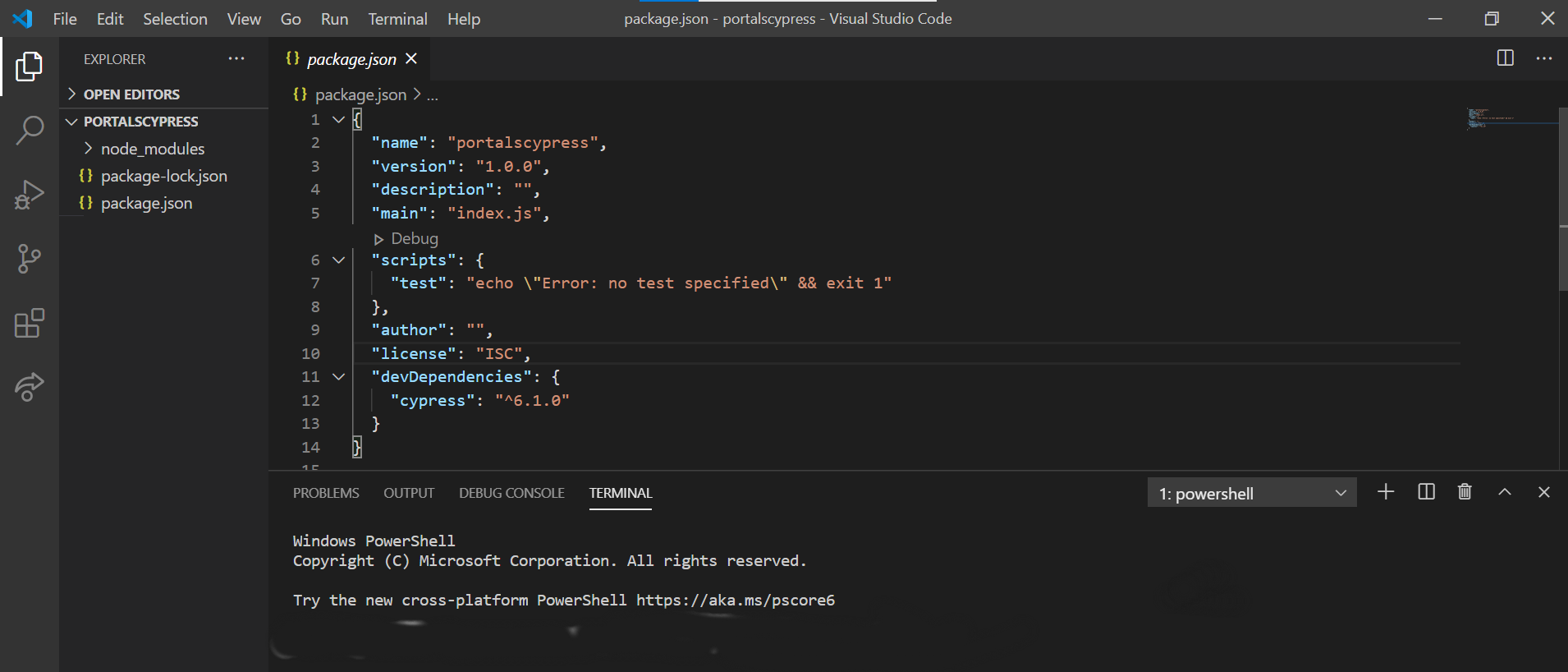
Cypress
In order to see what can we do with Cypress, we will use npx. Npx is installed alongside npm (Install the latest version of npm if you didn’t). This tool is a npm package runner that will help us to run cypress application.
npx cypress --help
As you can see there, we have the commands that we can use for cypress. However, we are going to use one that is going to be open
npx cypress open
First thing you are going to notice is that a new folder has appeared in your root called cypress, and a cypress.json has appeared as well.
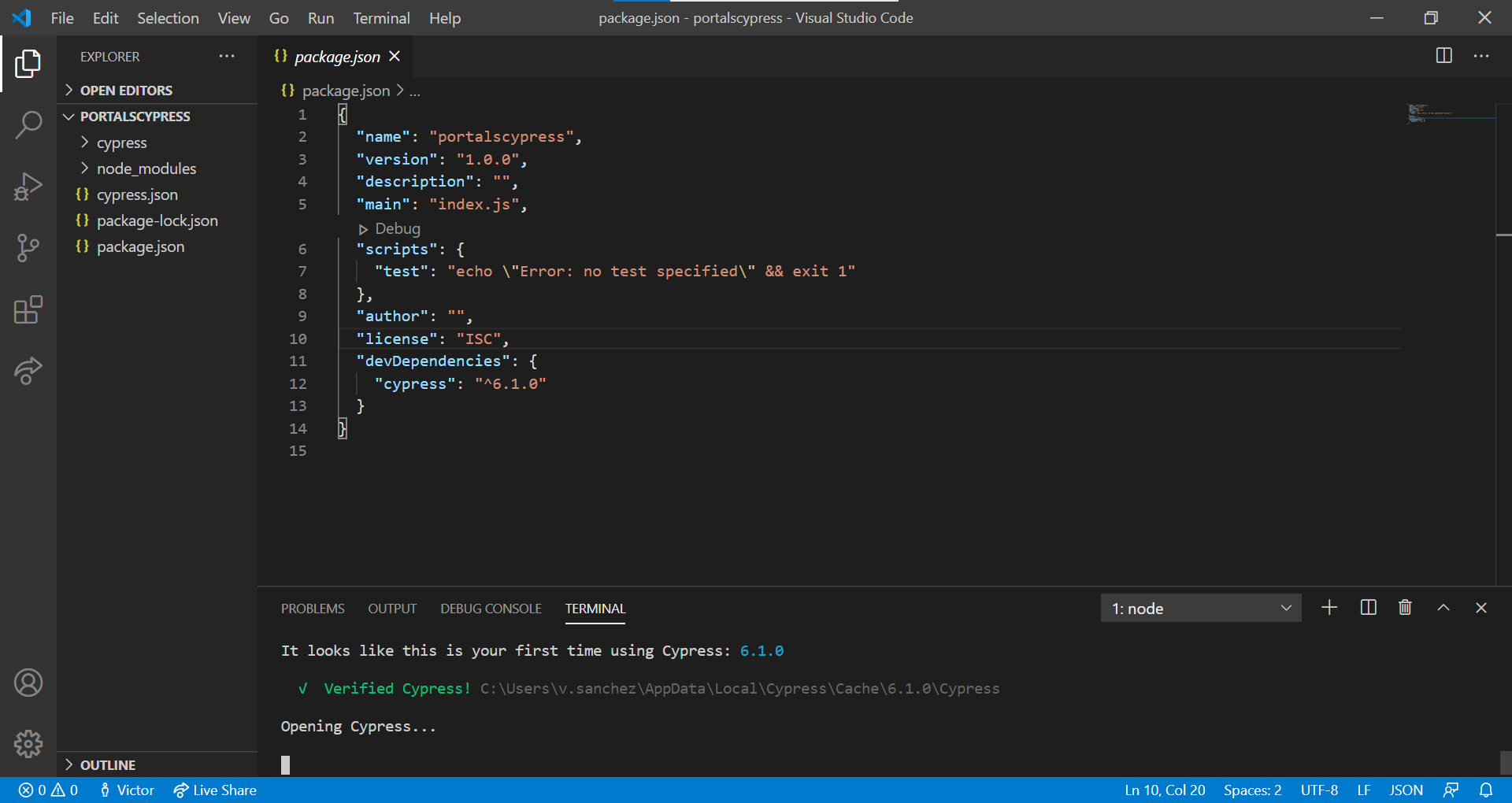
A new application is going to appear:
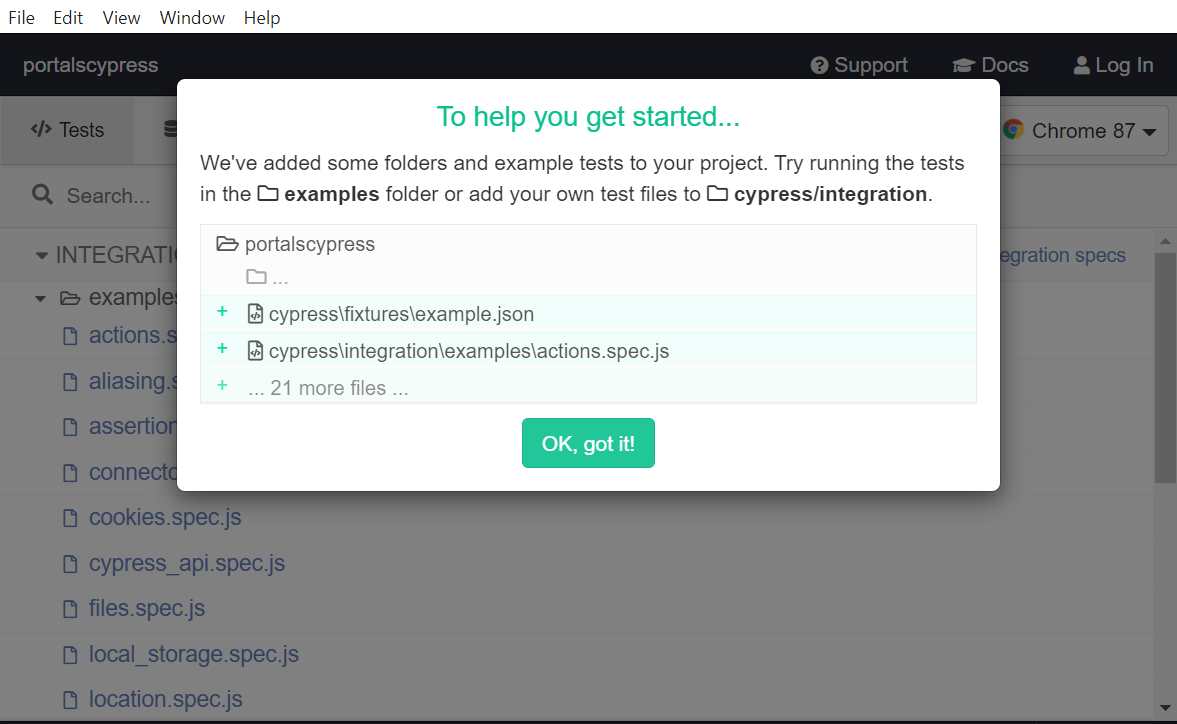
If we click the button, we will get he Integration Tests. Where is this coming from? Well, when I told you there was a new folder appearing with the name cypress, it came with some subfolders below it, and one of them is called integration/examples
You can take a look at them in order to see how the tests are performed. At the moment we will create a new folder called portals_testing and inside it we will create a file called my_first_cypress.js
In this folder we will write the following code:
describe("Portals Testing for log in", () => {
it("Logins correctly with user", () => {
cy.visit("yourpowerportals.powerappsportals.com/signin");
cy.get("#Username")
.type("myuser")
.should("have.value", "myuser");
cy.get("#Password")
.type("mysecretpassword")
.should("have.value", "mysecretpassword");
cy.get("button#submit-signin-local").click();
cy.url().should("include", "profile");
});
});
Once we save, we will see this in our “Cypress Application”: Image
We click in the test we want to run and this will be performed itself automatically.
As you can see, Cypress waits for each page to load, that’s awesome since in Portals you don’t know how long is going to take each page to load, that means you can perform your tests painless.
In the Cypress application we will see the following:
On the left, we have each step of the test we have performed, on the right, we have the actual browser embeded to see each iteration of the tests.
Let’s include another test:
describe("Portals Testing for log in", () => {
it("Logins correctly with user", () => { ... })
it("Logins wrongly", () => {
cy.visit("yourpowerportals.powerappsportals.com/signin");
cy.get("#Username")
.type("myuser")
.should("have.value", "myuser");
cy.get("#Password")
.type("wrongpassword")
.should("have.value", "wrongpassword");
cy.get("button#submit-signin-local").click();
cy.get("#loginValidationSummary").should(
"include.text",
"Invalid sign-in attempt"
);
});
});
In this last one, we are trying to sign in with a failing user and as you can see it’s in the same Describe, so it will perform the action after the first one.
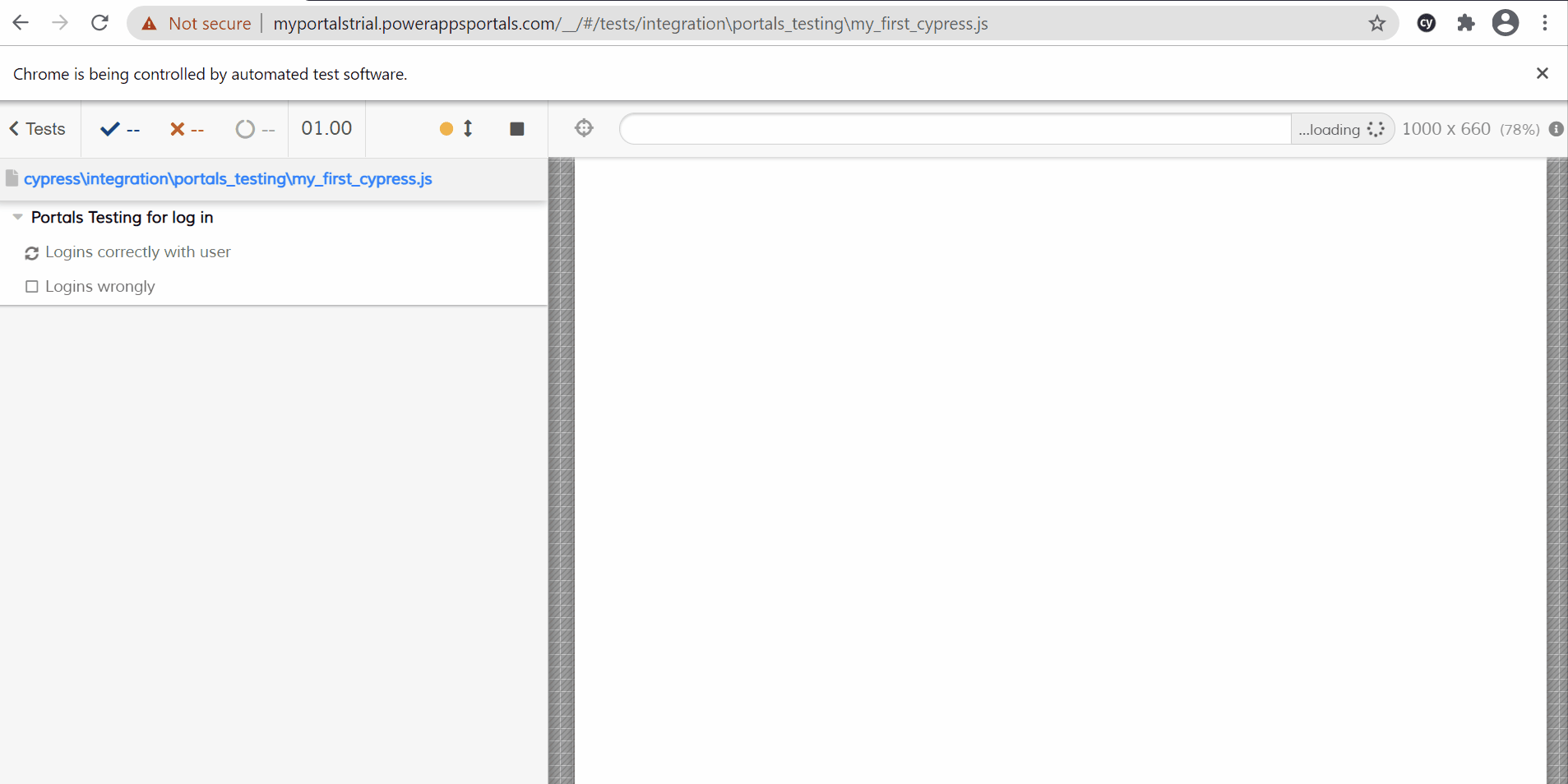
Items in the code
As you can see there are some items above that we should explain.
Describe. This will set our test name
it. Keyword in order to set a name to the specific task we want to test.
cy. Object bound to each test that contains several functions in order to get items…etc.
get. We can find the elements with a query selector.
should. The first parameter it has a quite range of values you can take, the second one refers to what’s the actual text you are expecting.
Conclusion
Inside Cypress you can find many objects and many events that are going to make your life easier. Probably I will do some videos about it and how we can perform a better testing with Cypress in our Portals with real examples, overall for the WebAPI.
As always, you can find me in LinkedIn or Twitter. As well, feel free to send me an email to me@victorsolaya.com if you want to discuss any of the content above.
I really hope you like this post. See you soon!